In this programming assignment you will simulate a regular Poker game otherwise known as the 5-Card Draw. Regular Poker is played with a standard deck of 52 cards. Cards are ranked from high to low in the following order: Ace, King, Queen, Jack, 10, 9, 8, 7, 6, 5, 4, 3, 2. $begingroup$ @RubberDuck, there are single deck Blackjack games still being played in Deadwood,SD at Saloon #10 I think it might be the only single deck game in Deadwood. (Last Time I was there to Play Blackjack) $endgroup$ – Malachi ♦ Aug 21 '14 at 15:09. And if you're a beginner in the programming world, than perhaps this tutorial will help you get a much better idea of how function, objects and DOM manipulation works in JavaScript along with HTML. While the following post won't be using any incredibly advanced topics in JavaScript, it is rather involved in what needs to go into a Blackjack game. 111B Assignment: Blackjack In the card game named 'blackjack' players get two cards to start with, and then they are asked whether or not they want more cards. Players can continue to take as many cards as they like. Their goal is to get as close as possible to a total of.

Purpose
The purpose of this assignment is to design and implement aJava program that simulates a solitaire version ofBlackJack. East windsor ct casino update today.
Partners
You may (and are encouraged to) work with at most one other partner on this assignment. You and your partner must be in the same lab section. Darmowe gry texas holdem poker. If you workwith a partner, submit one solution with both of your names on it.
Assignment
Create a BlueJ project named Program3 and placethe following files in it:
Your task is to modify the CardDeck and Card classessuch that a solitaire version of BlackJack (described below)can be simulated. The BlackJack class should be leftalone and not modified.
Blackjack Programming Assignment Questions
BlackJack Solitaire Background
Before the simulation is begun, the user will be askedto supply a lower bound between 1 and 21. Call thisvalue lowerBound.
Each time a game is simulated, the following stepsshould occur:
- Shuffle a regular, 52 card deck of cards.
- Deal the cards one at a time. If the total value of the cards dealt is ever greater than or equal to lowerBound and less than or equal to 21, the game is won. If the total value of the cards dealt ever exceeds 21, the game is lost.
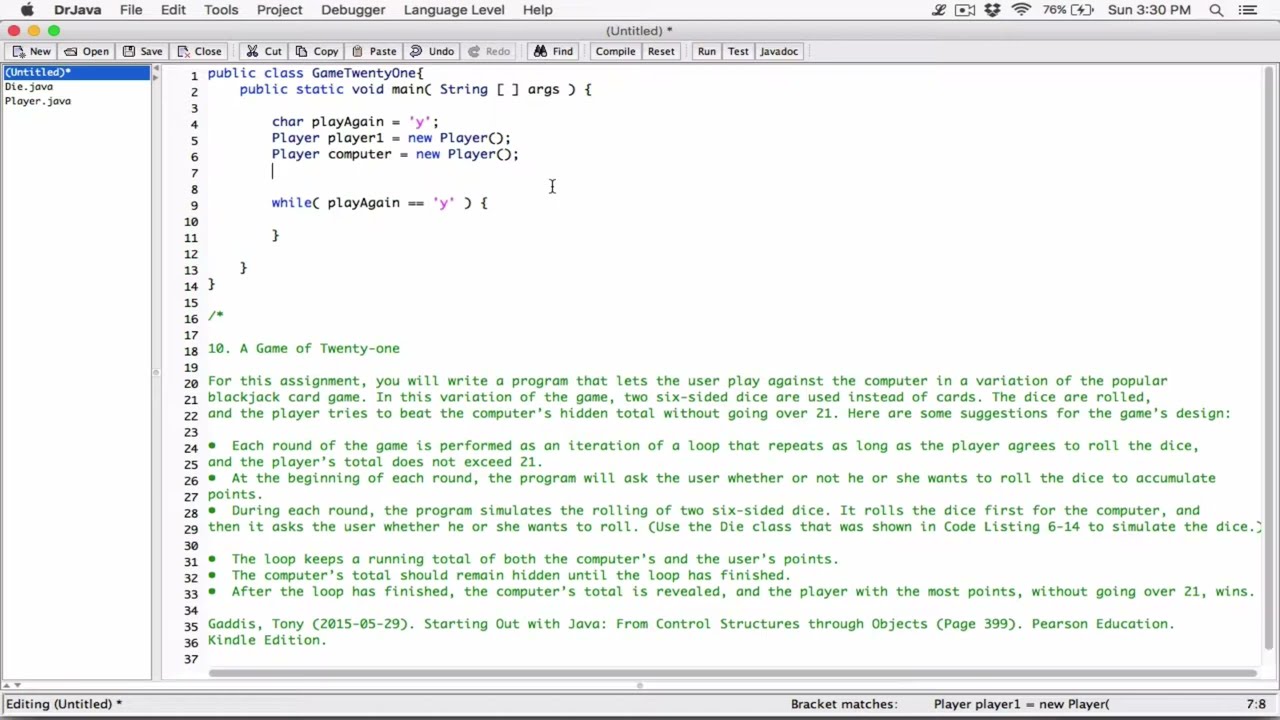
Purpose
The purpose of this assignment is to design and implement aJava program that simulates a solitaire version ofBlackJack. East windsor ct casino update today.
Partners
You may (and are encouraged to) work with at most one other partner on this assignment. You and your partner must be in the same lab section. Darmowe gry texas holdem poker. If you workwith a partner, submit one solution with both of your names on it.
Assignment
Create a BlueJ project named Program3 and placethe following files in it:
Your task is to modify the CardDeck and Card classessuch that a solitaire version of BlackJack (described below)can be simulated. The BlackJack class should be leftalone and not modified.
Blackjack Programming Assignment Questions
BlackJack Solitaire Background
Before the simulation is begun, the user will be askedto supply a lower bound between 1 and 21. Call thisvalue lowerBound.
Each time a game is simulated, the following stepsshould occur:
- Shuffle a regular, 52 card deck of cards.
- Deal the cards one at a time. If the total value of the cards dealt is ever greater than or equal to lowerBound and less than or equal to 21, the game is won. If the total value of the cards dealt ever exceeds 21, the game is lost.
In BlackJack, the value of a card is as follows:
- A two is worth 2 points.
- A three is worth 3 points.
- A four is worth 4 points.
- A five is worth 5 points.
- A six is worth 6 points.
- A seven is worth 7 points.
- An eight is worth 8 points.
- A nine is worth 9 points.
- A ten is worth 10 points.
- A jack is worth 10 points.
- A queen is worth 10 points.
- A king is worth 10 points.
- The first ace in the hand is worth 11 points, unless this ever makes the total value of the hand exceed 21 points. In this case, the first ace should be reevaluated to be worth 1 point. All subsequent aces are worth 1 point.
Getting Started
Blackjack Programming Assignment Examples
The three files that you are given will compile and run. Make surethat you understand them well before beginning. Notice that theCardDeck class contains the following methods that currentlydo nothing:
- initializeSimulation - the method should initialize appropriate instance variables before the BlackJack simulation begins.
- simulateBlackJack - the method should simulate one game of BlackJack solitaire and record whether the game was won or lost.
- getWins - the method should return the total number of times that BlackJack solitaire was won.
- getLosses - the method should return the total number of times that BlackJack solitaire was lost.
Grading (50 points possible)
- 5 points - the simulation works correctly for the range [1, 21].
- 5 points - the simulation works correctly for the range [11, 21].
- 5 points - the simulation works correctly for the range [17, 21].
- 5 points - the simulation works correctly for the range [18, 21].
- 5 points - the simulation works correctly for the range [19, 21].
- 5 points - the simulation works correctly for the range [20, 21].
- 5 points - the simulation works correctly for the range [21, 21].
- 5 points - CardDeck.java and Card.java contain appropriate comments, javadoc and otherwise.
- 5 points - the code that performs the simulation is reasonably efficient.
- 5 points - the code in CardDeck.java and Card.java is understandable and well written.
Blackjack Programming Assignment Example
Submission
Blackjack Programming Assignment Definition
E-mail the CardDeck.java and Card.javafiles as an attachment to your lab TA nolater than the start of your lab on Tuesday, October 28th.Late submissions receive no credit, but partial credit canbe earned by making an ontime submission.The subject of the e-mail should be CS 160, Program 3,your name, your partner's name.